Explore the world of web development through tutorials, best practices, and hands-on examples. Dive into frameworks, design principles, and coding challenges to level up your skills.
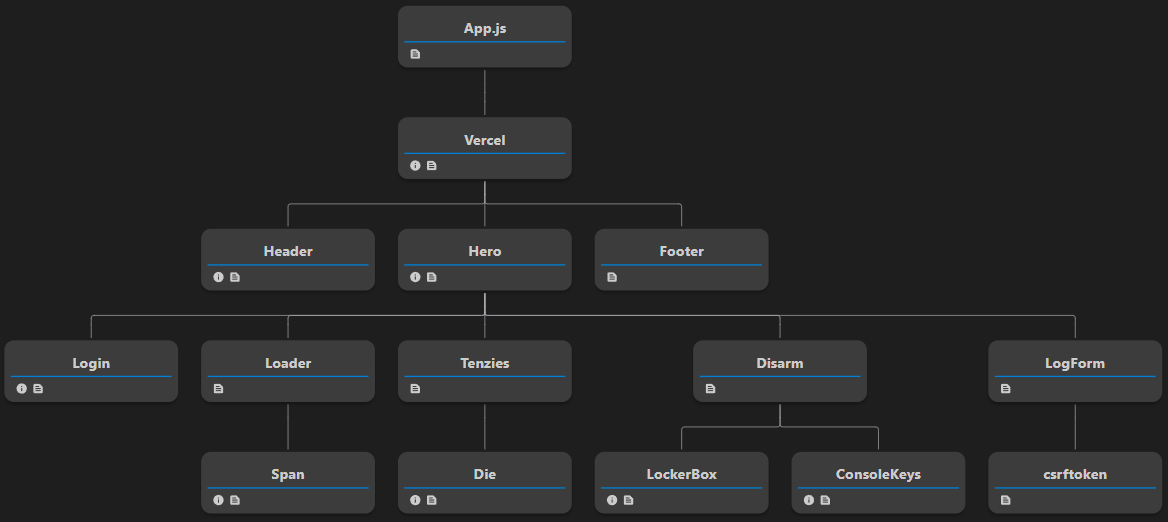
Table of contents [Show]
Mastering React Components: Building Dynamic UIs with Ease
Introduction
React has revolutionized front-end development by introducing a component-based architecture. Components are the building blocks of React applications, enabling developers to create reusable, modular, and maintainable user interfaces. In this guide, we'll explore the fundamentals of React components, their types, and how to use them effectively.
What Are React Components?
React components are self-contained units of code that describe a part of a UI. They can be functional (using functions) or class-based (using ES6 classes). Let's break down the two types:
1. Functional Components
// Simple functional component
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
// Usage
<Greeting name="Sarah" />
2. Class Components
// Class component with state
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
Increment
</button>
</div>
);
}
}
Key Concepts in React Components
Props: Passing Data Between Components
Props (short for properties) allow parent components to pass data to child components. They are read-only, ensuring a unidirectional data flow.
const UserCard = ({ username, email }) => (
<div className="user-card">
<h3>{username}</h3>
<p>{email}</p>
</div>
);
State: Managing Dynamic Data
State is used to manage data that changes over time. With React Hooks, functional components can now handle state:
import { useState } from 'react';
const ToggleButton = () => {
const [isOn, setIsOn] = useState(false);
return (
<button onClick={() => setIsOn(!isOn)}>
{isOn ? 'ON' : 'OFF'}
</button>
);
};
Example: Building a Todo List Component
const TodoList = () => {
const [todos, setTodos] = useState([]);
const [inputText, setInputText] = useState('');
const addTodo = () => {
if (inputText.trim()) {
setTodos([...todos, inputText]);
setInputText('');
}
};
return (
<div>
<input
type="text"
value={inputText}
onChange={(e) => setInputText(e.target.value)}
/>
<button onClick={addTodo}>Add Todo</button>
<ul>
{todos.map((todo, index) => (
<li key={index}>{todo}</li>
))}
</ul>
</div>
);
};
Conclusion
React components empower developers to build scalable and dynamic applications. By mastering props, state, and lifecycle methods (like useEffect
), you can create UIs that are both efficient and easy to maintain. Start small, experiment with component composition, and soon you'll be crafting complex interfaces with confidence!